Difference between Full and Complete Binary Tree
A binary tree is a type of data structure where each node can only have two offspring at most named as “left” and “right” child.
A Binary Tree
There are different types of binary tree but here we are going to discuss about the difference of Complete binary tree and Full binary tree.
Full Binary Tree:
A full binary tree is a binary tree in which all of the nodes have either 0 or 2 offspring. In other terms, a full binary tree is a binary tree in which all nodes, except the leaf nodes, have two offspring.
A Full Binary Tree
Let, i be the number of internal nodes
n be the total number of nodes
l be number of leaves
λ be number of levelsThen,
The number of leaves is (i + 1).
The total number of nodes is (2i + 1).
The number of internal nodes is (n – 1) / 2.
The number of leaves is (n + 1) / 2.
The total number of nodes is (2l – 1).
The number of internal nodes is (l – 1).
The number of leaves is at most (2λ – 1).
Complete Binary Tree:
A binary tree is said to be a complete binary tree if all its levels, except possibly the last level, have the maximum number of possible nodes, and all the nodes in the last level appear as far left as possible.
A Complete Binary Tree
There are 2 points that you can recognize from here,
- The leftmost side of the leaf node must always be filled first.
- It isn’t necessary for the last leaf node to have a right sibling.
Check the following examples to understand the full and complete binary tree in a better way.
Example 1:
Neither complete nor full
- Node C has just one child therefore, it is not a Full binary tree.
- Node C also has a right child but no left child, therefore it is also not a Complete binary tree.
Hence, the binary tree shown above is neither complete nor full binary tree.
Example 2:
Full but not complete
- All of the nodes have either 0 or 2 offspring, therefore, it is a Full binary tree.
- It is not a Complete binary tree because node B has no children whereas node C has children, and according to a complete binary tree, nodes should be filled from the left side.
Hence, the binary tree shown above is a Full binary tree and it is not a Complete binary tree.
Example 3:
Complete but not full
- It is a complete binary tree as all the nodes are left filled.
- Node B has just one child, therefore, it is not a full binary tree.
Hence, the binary tree shown above is a Complete binary tree and it is not a Full binary tree.
Example 4:
Complete and full
- It is a Complete binary tree because all the nodes are left filled.
- All of the nodes have either 0 or 2 offspring, therefore, it is a full binary tree.
Hence, the binary tree shown above is both a complete and a full binary tree.
S. No. | Complete Binary Tree | Full Binary Tree |
1. | In a complete binary tree, a node in the last level can have only one child. | In a full binary tree, a node cannot have just one child. |
2. | In a complete binary tree, the node should be filled from the left to right. | There is no order of filling nodes in a full binary tree. |
3. | Complete binary trees are mainly used in heap-based data structures. | Full binary tree has no application as such but is also called a proper binary tree. |
4. | A complete binary tree is also called almost complete binary tree. | A full binary tree also called proper binary tree or 2-tree. |
S.NO | B-tree | Binary tree |
---|---|---|
1. | In a B-tree, a node can have maximum ‘M'(‘M’ is the order of the tree) number of child nodes. | While in binary tree, a node can have maximum two child nodes or sub-trees. |
2. | B-tree is called a sorted tree as its nodes are sorted in inorder traversal. | While binary tree is not a sorted tree. It can be sorted in inorder, preorder, or postorder traversal. |
3. | B-tree has a height of log(M*N) (Where ‘M’ is the order of tree and N is the number of nodes). | While binary tree has a height of log2(N) (Where N is the number of nodes). |
4. | B-Tree is performed when the data is loaded into the disk. | Unlike B-tree, binary tree is performed when the data is loaded in the RAM(faster memory). |
5. | B-tree is used in DBMS(code indexing, etc). | While binary tree is used in Huffman coding and Code optimization and many others. |
6. | To insert the data or key in B-tree is more complicated than a binary tree. | While in binary tree, data insertion is not more complicated than B-tree. |
7. | B-tree is a self-balancing tree. The height of the tree is automatically adjusted on each update. | A binary tree is not a self-balancing tree. |
Comparison of Searching methods in Data Structures
the basic differences between two searching techniques, the sequential search and binary search.
Sequential Search | Binary Search |
---|---|
Time complexity is O(n) | Time complexity is O(log n) |
Finds the key present at first position in constant time | Finds the key present at center position in constant time |
Sequence of elements in the container does not affect. | The elements must be sorted in the container |
Arrays and linked lists can be used to implement this | It cannot be implemented directly into the linked list. We need to change the basic rules of the list to implement this |
Algorithm is iterative in nature | Algorithm technique is Divide and Conquer. |
Algorithm is easy to implement, and requires less amount of code. | Algorithm is slightly complex. It takes more amount of code to implement. |
N number of comparisons are required for worst case. | Log n number of comparisons are sufficient in worst case. |
Difference between Binary Search Tree and AVL Tree
Binary Search Tree:
A binary Search Tree is a node-based binary tree data structure that has the following properties:
- The left subtree of a node contains only nodes with keys lesser than the node’s key.
- The right subtree of a node contains only nodes with keys greater than the node’s key.
- The left and right subtree each must also be a binary search tree.
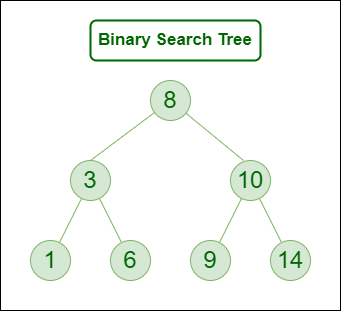
Binary Search Tree
AVL Tree:
AVL tree is a self-balancing Binary Search Tree (BST) where the difference between heights of left and right subtrees for any node cannot be more than one.
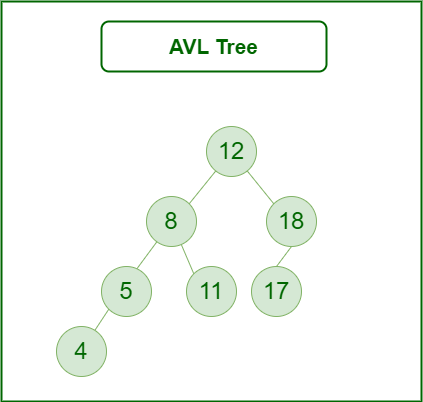
AVL Tree
Difference between Binary Search Tree and AVL Tree
Binary Search Tree | AVL Tree |
---|---|
In Binary Search Tree, every node does not follow the balance factor. | In AVL Tree, every node follows the balance factor i.e. 0, 1, -1. |
Every Binary Search tree is not an AVL tree. | Every AVL tree is a Binary Search tree. |
Simple to implement. | Complex to implement. |
The height or depth of the tree is O(n). | The height or depth of the tree is O(logn) |
Searching is not efficient when there are a large number of nodes in the Binary Search tree. | Searching is efficient because searching for the desired node is faster due to the balancing of height. |
The Binary Search tree structure consists of 3 fields left subtree, data, and right subtree. | AVL tree structure consists of 4 fields left subtree, data, right subtree, and balancing factor. |
It is not a balanced tree. | It is a balanced tree. |
In Binary Search tree. Insertion and deletion are easy because no rotations are required. | In an AVL tree, Insertion and deletion are complex as it requires multiple rotations to balance the tree. |
Red Black Tree vs AVL Tree
Red Black Tree:
Properties:
- Self-Balancing is provided by painting each node with two colors(Red or Black).
- When the Tree is modified, a new tree is subsequently rearranged and repainted.
- It requires 1 bit of color information for each node in the tree.
- Time complexity: O(logn).
Constraints maintained by Red Black Tree:
- Root is always black.
- All NULL leaves are black, and both children of a red node are black.
- Every simple path from a given node to any of its descendant leaves contains the same number of black
nodes. - Path from root to farthest leaf is no more than twice as long as the path from the root to nearest leaf.
- Time complexity: O(logn).
AVL(Adelson-Velskii and Landis) Tree
Properties:
- Height difference of the left and right subtree of the node should be less than 2.
- Re-balancing is done when the heights of two child subtrees of a node differ by more than one.
- Faster retrievals as strictly balanced.
Difference:
Basis of comparison | Red Black Trees | AVL Trees |
---|---|---|
Lookups | Red Black Trees has fewer lookups because they are not strictly balanced. | AVL trees provide faster lookups than Red-Black Trees because they are more strictly balanced. |
Colour | In this, the color of the node is either Red or Black. | In this, there is no color of the node. |
Insertion and removal | Red Black Trees provide faster insertion and removal operations than AVL trees as fewer rotations are done due to relatively relaxed balancing. | AVL trees provide complex insertion and removal operations as more rotations are done due to relatively strict balancing. |
Storage | Red Black Tree requires only 1 bit of information per node. | AVL trees store balance factors or heights with each node thus requiring storage for an integer per node. |
Searching | It does not provide efficient searching. | It provides efficient searching. |
Uses | Red-Black Trees are used in most of the language libraries like map, multimap, multiset in C++, etc. | AVL trees are used in databases where faster retrievals are required. |
Balance Factor | It does not gave balance factor | Each node has a balance factor whose value will be 1,0,-1 |
Balancing | Take less processing for balancing i.e.; maximum two rotation required | Take more processing for balancing |